I ran into an issue the other day where my UpdateProgress control (an animated GIF) would display behind any div that was using the DropShadowExtender control from the AJAX Control Toolkit. In order to fix this issue I set the CSS class on the div of the UpateProgress to "postion: fixed" and the "z-index" to an arbitrarily high number.
This is before the CSS change. Notice the animated GIF just peeking out from the end of the drop shadow at the bottom right of this image.

And here is what it looks like after the CSS change. The animated GIF now displays properly in front of the div using the drop shadow.
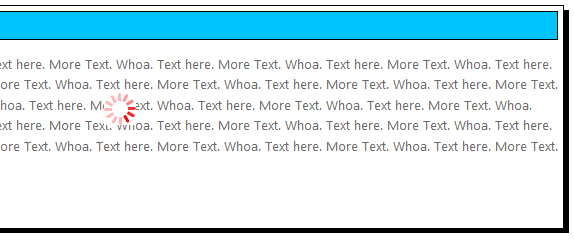
CSS:
div.divProgress { z-index: 1001; position: fixed; }
ASPX:
<%@ Register Assembly="AjaxControlToolkit" Namespace="AjaxControlToolkit" TagPrefix="ajaxtk" %>
<ajaxtk:DropShadowExtender id="dse" runat="server" TargetControlID="divPanel"
TrackPosition="true" />
<div id="divPanel" runat="server" class="Box">
<div class="BoxHeader">This Is My Header</div>
<div>
<p>Text here. More Text. Whoa. Etc...</p>
<p>
<asp:Button ID="btnDoSomething" runat="server" Text="Do Something"
onclick="btnDoSomething_Click" />
</p>
</div>
</div>
<ajaxtk:AlwaysVisibleControlExtender ID="AlwaysVisibleControlExtender" runat="server"
TargetControlID="updateProgress" HorizontalSide="Center" VerticalSide="Middle" />
<asp:UpdateProgress ID="updateProgress" runat="server" DynamicLayout="true" >
<ProgressTemplate>
<div class="divProgress" id="divProgress" runat="server" align="center">
<img src="~/images/red_rotation.gif" id="imgUpdateProgress"
runat="server" alt="Please Wait..." />
</div>
</ProgressTemplate>
</asp:UpdateProgress>
I got this error in Visual Studio when I was running one of our projects on a new development machine where I had just recently installed a fresh copy of Visual Studio 2008. After installing the Microsoft .NET SDK this error went away. Hopefully this post helps someone else out there.
This one is simple but nice to know. To remove a control from the tab order you can just set its TabIndex to -1. I thought there might be a "turn tabbing off" property, but this seems to be the easiest way.
<asp:CheckBox ID="myCB" runat="server" Text="MyCB" TabIndex="-1" />
NOTE: If you set your LinqDataSource's AutoPage property to true and are using SQL Server (2005+ I believe) then it will automatically use Skip() and Take() as seen below. However, if you want to do it manually or wonder how it works then read on.
Creating a GridView (or other data control) with efficient paging is very easy with LINQ. You can thank the Take and Skip operators for this; they allow you to only pull back the records you need. In the simple example below we are using a LinqDataSource and handling its onselecting method to create our LINQ query and do our paging. Set AutoPage to false since we are writing code to handle paging ourselves. Also the PageSize property of the GridView control is being populated from a constant in the code-behind class.
ASPX:
<asp:LinqDataSource ID="linqDS" runat="server" AutoPage="false"
ContextTypeName="Namespace.MyDataContext"
onselecting="linqDS_Selecting" />
<asp:GridView ID="myGV" runat="server" DataSourceID="linqDS"
AllowPaging="true" PageSize="<%# PAGE_SIZE %>"
AutoGenerateColumns="false">
<Columns>
<!--Removed for simplicity -->
</Columns>
</asp:GridView>
Code-behind:
// Const declared at top of code-behind
public const int PAGE_SIZE = 100;
protected void linqDS_Selecting(object sender, LinqDataSourceSelectEventArgs e)
{
// LINQ query
var query = from c in myDC.Class
select c;
// Do advanced query logic here (dynamically build WHERE clause, etc.)
// Set the total count
// so GridView knows how many pages to create
e.Arguments.TotalRowCount = query.Count();
// Get only the rows we need for the page requested
query = query.Skip(myGV.PageIndex * PAGE_SIZE).Take(PAGE_SIZE);
e.Result = query;
}
As you probably know, you can implement caching in an ASPX page using the OutputCache directive. However, you can't use this in an ASHX handler. So what if you are using a handler to server binary files from the database and you want to improve peformance by using server side output caching? You'll just need to add some of your own caching logic in your code-behind. Here is some sample code of how you can do this. In this very basic example we are passing the ID of our object on the query string.
// Get ID from query string
int myID = Convert.ToInt32(context.Request.QueryString["ID"]);
// Attempt to get item from cache if it exists
string myCacheKey = "MyKey" + myID; // Unique Identifier
MyObject myObject = context.Cache[myCacheKey] as MyObject;
if (myObject == null)
{
// Item isn't cached; Grab business object from the database
myObject = MyObject.GetMyObject(myID);
// Add object to Cache using the cache key
// In this example we are caching for 30 minutes
context.Cache.Insert(myCacheKey, myObject,
null, DateTime.UtcNow.AddMinutes(30),
Cache.NoSlidingExpiration);
}
// Code to generate file from binary data here
Today I had a problem hitting a breakpoint in an .ASHX file I created. I noticed the project was using the built-in Visual Studio Development Server. Once I switched over to using IIS I had no problem hitting the breakpoint. I thought it was very strange, but hopefully this might help someone else out if they manage to find this post via Google.
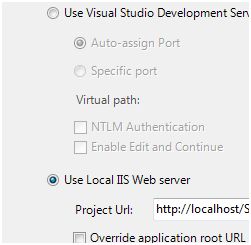
We saw the above message in SourceSafe after moving to SQL Server 2008 and then saving the scripts for our tables back to SourceSafe. It appears that SQL 2008 Management Studio uses a different text encoding than prior versions of Management Studio/Enterprise Manager.

To save your script using a specific text encoding. You can "Save as.." and then there is an arrow to the right of the Save button. Click this and select "Save with Encoding...". Then you can select the specific encoding you need. In my case I needed to change the encoding from "Western Eurpean (Windows) - Codepage 1252" to "Unicode - Codepage 1200".

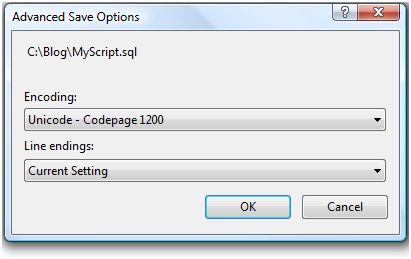
Below is the syntax you can use in the Properties window of the LINQ Designer to map to an enum. Set this under the Type property and use the fully qualified name of your enum.
Example: global::MyClassLibrary.MyStuff.MyClassName
Screenshot:
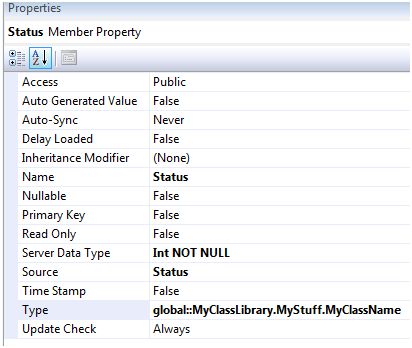
I tend to look this up quite a bit, so here it is for quick reference. This converts a datetime column into a MM/DD/YYYY format.
UPDATE MyTable
SET DateColumn2 = CONVERT(varchar(10), DateColumn1, 101)
I just wanted to post that I successfully installed my old Turtle Beach Santa Cruz sound card in Windows 7 (32-bit). If you look on the Turtle Beach website they say Vista and Windows 7 are not supported. I tried installing the latest drivers for Windows XP anyway. These drivers are named sc_4193.exe and you can find them here on Turtle Beach's website. After the setup wizard completed I got a message saying device installation was not successful. However, after restarting the audio seems to be working fine.
However, as Rob pointed out in the comments below, much of the functionality in the Santa Cruz Control Panel does not appear to work, most notably the Graphic Equalizer settings.
I just thought I would post this in case anyone was thinking about trying to install their old sound card in Windows 7.
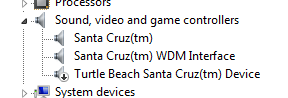
Note: The disabled device in the image above is just the gameport on the sound card.